Tuesday, December 1, 2009
.NET Authentication Process for Server
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
//using [Web Service Name];
public partial class _Default : System.Web.UI.Page
{
public DataSet ds_GetPickUpDropOffInDataSet = new DataSet();
protected void Page_Load(object sender, EventArgs e)
{
try
{
ParveenAPIService pas = new ParveenAPIService();
Authentication auth = new Authentication();
auth.UserId = "1023";
auth.UserName = "hema";
auth.Password = "54321";
pas.AuthenticationValue = auth;
ds_GetPickUpDropOffInDataSet = pas.GetPickUpDropOffInDataSet();
//The HTMLEncode method applies HTML encoding to a specified string.
//DataSet..::.GetXml Method returns the XML representation of the data
//stored in the DataSet.
Response.Write(Server.HtmlEncode(ds_GetPickUpDropOffInDataSet.GetXml()));
}
catch (Exception err)
{
Response.Write(err);
}
}
}
.NET Client and Server [HTTP Request and Response]
//Adding Namespace
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Collections.Specialized;
using System.Reflection;
using System.Net;
using System.IO;
public partial class _Default : System.Web.UI.Page
{
public string url = null;
public NameValueCollection coll;
protected void Page_Load(object sender, EventArgs e)
{
try
{
url = "http://localhost:____.aspx";
System.Net.HttpWebRequest webrequest = (System.Net.HttpWebRequest)System.Net.HttpWebRequest.Create(url);
webrequest.Method = "GET";
System.Net.WebHeaderCollection headers = new System.Net.WebHeaderCollection();
headers.Add("pvalue", "12345");
//Adding header name and value to a URL
webrequest.Headers = headers;
System.Net.HttpWebResponse webResponse = (System.Net.HttpWebResponse)webrequest.GetResponse();
// Get the response stream
StreamReader reader = new StreamReader(webResponse.GetResponseStream());
// Read the whole contents and return as a string
string result = reader.ReadToEnd();
//Print the contents
Response.Write(result);
}
catch (Exception err)
{
Response.Write(err);
}
}
}
Server Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
//using [Web Service Name];
using check; //check is namespace name
public partial class _Default : System.Web.UI.Page
{
public string str_GetPickUpDropOffInString = null;
protected void Page_Load(object sender, EventArgs e)
{
//GetPickUpDropOffInString()
try
{
// Authentication Process
ParveenAPIService pas = new ParveenAPIService();
Authentication auth = new Authentication();
auth.UserId = "1026";
auth.UserName = "hema";
auth.Password = "54321";
pas.AuthenticationValue = auth;
//Checking for private key
//Creating an object for class
Check_Class pkobj = new Check_Class();
//Accessing the method Check_Code using the object pkobj and storing it in a string.
//Request.Headers["pvalue"] is for requesting the header's
//value by specifying the header name.
string pk=pkobj.Check_Code(Request.Headers["pvalue"].ToString());
if (pk == "True") //Private key specified
{
str_GetPickUpDropOffInString = pas.GetPickUpDropOffInString();
Response.Write(str_GetPickUpDropOffInString);
}
else
{
Response.Write("Invalid User");
}
}
catch (Exception err)
{
Response.Write(err);
}
}
}
Tuesday, October 27, 2009
Code to connect PHP and MySQL
$con = mysql_connect("localhost","root","root");
echo "connected";
if (!$con)
{
die('Could not connect: ' . mysql_error());
}
Program to create database and create table:
// create connection
$con = mysql_connect("localhost","root","root");
if (!$con)
{
die('Could not connect: ' . mysql_error());
}
// Create database
if (mysql_query("CREATE DATABASE employee",$con))
{
echo "Database created";
}
else
{
echo "Error creating database: " . mysql_error();
}
// Create table
mysql_select_db("employee", $con);
$sql = "CREATE TABLE emp
(
ename varchar(15),
eno int,
age int
)";
// Execute query
mysql_query($sql,$con);
mysql_close($con);
Program to insert values to table:
// create connection
$con = mysql_connect("localhost","root","root");
if (!$con)
{
die('Could not connect: ' . mysql_error());
}
// select database
mysql_select_db("employee", $con);
mysql_query("INSERT INTO emp (ename,eno,age)
VALUES ('Sam', '1001', '24')");
mysql_query("INSERT INTO emp (ename,eno,age)
VALUES ('Sunil', '1002', '32')");
mysql_close($con);
Sunday, October 18, 2009
Receive SMS When Someone IM’s You (GMAIL)
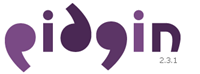
The SMS, is sent via Google Calendar,the SMS doesn’t give you the actual text of the message. It gives just the name of the contact and the date / time the message was sent to you. If multiple contacts leave messages, they will be all bundled together and sent to you in one SMS.
Here’s how to get this working
•Pidgin installed on your computer.
•A Google account (with SMS reminders enabled on Google Calendar).
•Google supported mobile phone network to receive SMS.
•Your PC synched with NTP (Network Time Protocol).
Installation :
For Windows Users :
Download the plug-in and place the “gsms.dll” file in the Pidgin plugins folder of Pidgin on your PC. Then open Pidgin, go to “tools” menu then “plugins” and activate the plugin.
For Linux Users :
copy gsms.so to ~/.purple/pluginsConfiguration
Screenshot :
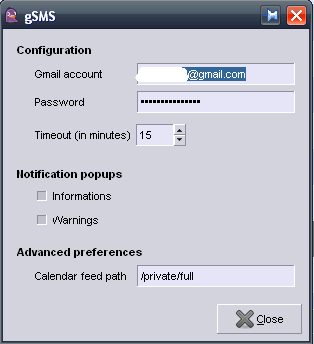
Configuration Settings:
Gmail account: your gmail e-mail (example: xyz@gmail.com).
Password: password for specified account.
Timeout: Number of minutes for gsms to collect chats until it sends them.
Calendar feed path: Which calendar to use (example: “/private/full” translates to: http://www.google.com/calendar/feeds/xyz@gmail.com/private/full)
Thank you.
HEMA @ HELOISE
How to Install MySQL 5.x on Windows XP Pro
http://dev.mysql.com/downloads/ ( MySQL Community Server - Current Release - Windows Essentials)
Double click it and press Run to start the install
Press Next
Select Typical
Press Next
Press Install to install to C:\Program Files\MySQL\MySQL Server 5.x
Press Next
Press Next
Press Finish to Configure the MySQL Server
Press Next to to start the Configuration Wizard
Select Standard Configuration and Press Next
Check both boxes, Install As Windows Service and Include Bin Directory
Press Next
Add a root password if you wish or else uncheck Modify Security Settings.
If you do add a root password, once you install the Personal Server, you will have to edit the Personal Server config file to add the password. The config file will be C:\Inetpub\wwwroot\cinemapremiereps\configdb.php (or
You can open configdb.php with notepad and add your password in the db_password field between the single quotes.
Save the file.
Press Next
Press Execute
Press Finish to close the Wizard
Once you have installed the web server, php, MySQL, and the personal server, you should reboot your computer before testing the movie manager.
How to Install PHP 5.x on Windows XP Pro
To unzip using Windows default Extract Wizard
right click the zip file
select Extract All...
click Next
browse to My Computer > SYSTEM(C:)
with SYSTEM(C:) highlighted, click Make New Folder
enter php
press OK
in the ...extract to this directory. field you should see C:\php
press Next
then press Finish
To unzip using WinZip
right click the zip file
select Extract to...
select SYSTEM(C:) in the Folders/drives: window
with SYSTEM(C:) highlighted, click New Folder
enter php
press OK
in the Extract To: field you should see C:\php
press Extract
Add c:\php to your Environment Variables
right click 'My Computer' on desktop
select 'Properties' -> 'Advanced'
select 'Environment Variables' at the bottom of the screen
in 'System variables', scroll down to select 'Path' and press 'Edit'
add ;C:\php at the end of the 'Variable value:' field (variable values need to be separated by ;)
press Ok, press Ok, press Ok.
Add the .php extension
For IIS, add it to the Application Mappings.
Start -> Control Panel -> (Performance and Maintenance) ->Administrative Tools -> Internet Information Services
Expand your local computer by clicking the +
Right click on Web Sites
Select Properties
Select the Home Directory tab
Press the Configuration button on the lower right side of the screen
Press the Add button
Browse to C:\php
Change Files of type: at the bottom of the screen to Dynamic Link Libraries (*.dll)
Double click on php5isapi.dll or select php5isapi.dll and press Open
Enter .php in the Extension field (be sure to enter the '.' before the php)
Press OK
Press Apply
Press OK
Press OK
Close the Internet Information Services screen
For Abyss,
Declare the interpreter
Open Abyss Web Server's console. In the Hosts table, press Configure in the row corresponding to the host to which you want to add PHP support.
Select Scripting Parameters.
Check Enable Scripts Execution.
Press Add in the Interpreters table.
In the Interpreter field, press Browse..., go to the directory where you have installed PHP and click on php5isapi.dll .
Set Type to PHP Style.
Check Use the associated extensions to automatically update the Script Paths.
Press Add in the Associated Extensions table.
Enter php in the Extension field and press OK.
Press OK
Press Add in the Custom Environment Variables table.
Enter REDIRECT_STATUS (with no leading or trailing spaces) in the Name field and 200 in the Value field and press OK.
Press OK in the Scripting Parameters dialog.
Declare new index files
Select Index Files.
Press Add in the Index Files table.
Enter index.php in the File Name field and press OK.
Apply the modifications
Press Restart to restart the server.
For Apache, in the Apache install folder (C:\Program Files\Apache Group\Apache2 if the default was chosen):
open the conf folder
open the httpd.cnf file with notepad or some other text editor
Add:
LoadModule php5_module "C:/php/php5apache2.dll"
Addtype application/x-httpd-php .php
You may not need to do this step, but if you have a problem running php files you may need to come back and configure the path to php.ini
Search for PHPIniDir and set it to "C:/windows"
If PHPIniDir is not there, you can add it: PHPIniDir "C:/windows" or whatever your windows directory is.
Save the file and restart the Apache server by clicking on the Apache icon in the system tray, moving your cursor over Apache2 and selecting Restart.
Copy Cinema Premiere's modified php.ini to your Windows directory which should be either C:\WINDOWS or C:WINNT depending on how you initially installed Windows XP Pro.
Cinema Premiere's modified php.ini can either be downloaded from here or
extracted from either the CinemaPremierePSTest.zip or the CinemaPremierePS.zip
right click either CinemaPremierePSTest.zip or CinemaPremierePS.zip
select Extract to...
in the Files area, select Files: and type in php.ini
in the Folders/drives window, expand SYSTEM (C:) by selecting the + in front of SYSTEM (C:)
select your Windows directory (either WINDOWS or WINNT)
select Extract
Thank you friends.
HEMA @ HELOISE
Configuring and Deploying PHP
Let’s get started. Since you are installing on a Windows computer, you will most likely be using IIS for a web server, however, it is not required. You must have some web server running however, and you are quite welcome to use Apache or another web server of your choice, however, since that falls outside the scope of this topic i will leave that discussion for another day.
Here i have used php-5.3.0-nts-Win32-VC9-x86.msi setup file for PHP and IIS for server.
You can install from this link.
- PHPphp5isapi.dll
- php5ts.dll
Some things to know about IIS:
IIS v5.1 is included with Windows 2000 Professional, and Windows XP Professional, but is not installed by default. (*note – IIS 5.1 is not included with XP Home edition and is not available as a stand alone download from Microsoft. If you are an XP Home user, you will need to look for an alternative web server solution.) Windows 2000 server includes IIS 5.1, and Windows 2003 server includes IIS 6.
If you haven’t already done so begin by installing IIS. To do this go to Control Panel Add/Remove Programs Add/Remove Windows Components. In the dialogue box that comes up, check the option for Internet Information Services(IIS) and click the “Details” button. Select any additional features that would like to have installed and click OK. Click OK again to begin the installation. This will install IIS and start the web server service automatically. You should not need to reboot. To test your installation open your web browser and type in http://localhost/ . This should bring up the default IIS page. If it does, your installation was a success and you can immediately get started on installing PHP.
PHP Installation & Settings:
The first step is to extract all of the files from the downloaded zip file or install the PHP setup into C:PHP (create the folder if it doesn’t already exist).
You may choose a different location, although it is not recommended. The path must NOT have spaces, for example, you cannot use C:Program FilesPHP. Some web servers may not be able to handle the path name and will fault. PHP 5 includes a CGI executable, a CLI executable as well as the server modules. The DLLs needed for these executables can be found in the root of the PHP folder (C:PHP). php5ts.dll needs to be available to the web server. To do this, you have 3 options:
1. Copy php5ts.dll to the web server’s directory (C:inetpubwwwroot).
2. Copy php5ts.dll to the Windows system directory (C:windowssystem32).
3. Add the PHP directory path to the environment variables PATH.
We will go with option 3, because we would like to keep all of our PHP install files in the same location, for easier cleanup later, if needed. Let’s proceed…
Instructions on how to put C:php in environment variables PATH:
1. Right-Click on My computer and choose “properties”.
2. Go to Control Panel, and select “System”.
Once here, we want to select the Advanced tab. In the Advanced tab, click the “Environment Variables” button. There are two sections in the Environment Variables window, User Variable and System Variables. We will be using System Variables. Scroll down in System Variables until you find the variable PATH. Highlight that line and the select Edit below the System Variables window. We will only be adding to the Variable Value. BE CAREFUL HERE. You do not want to delete anything on this line. Simply find the end of the line and add a semi-colon ( ; ) if there is not one already. After the semi-colon, type: C:PHP and then hit OK. Now click OK on the Environment Variables window. Finally click OK on the System Properties window and we are done with this part.
Now we must restart the computer to make the Environment Variables changes come into play. We cannot simply log off and log on, you must restart.
The next step is to set up a config file for PHP, php.ini. In C:PHP you will find two files named php.ini-dist and php.ini-recommended. We will use php.ini-recommended for this install, and all you need to do is rename it from php.ini-recommended to php.ini.
1. doc_root = C:inetpubwwwroot
2. cgi.force_redirect = 0
Now PHP is installed, lets move on to preparing our IIS to use PHP.
Configure IIS to use PHP:
1. Open IIS
2. Under Home Directory: Set “Execute Permissions” to “Scripts Only”
3. Click on configuration..
a. Click Add
b. Set “executable” to C:PHPphp5isapi.dll
c. Set “extension” to .php (don’t forget to include the . )
d. Click OK
e. Click Apply, then OK.
Under ISAPI Filters:
a. Click “Add”
b. Set Filter Name to PHP
c. Set Executable to C:PHPphp5isapi.dll
d. Click OK.
e. Click Apply, then OK.
Restart the Web Server
Now we want to test PHP on our system. To do this, we will create a file called phpinfo.php and it will be used to display all of the PHP info from our system in our web browser.
1. Open Notepad and type:
2. Save the file as phpinfo.php and select the file type ‘All Files’ (Important: do not save the file as .txt, as it will not work).
3. Move the file into C:inetpubwwwroot
4. Open your web browser and type: http://localhost/phpnfo.php
5. Your browser should display a lot of PHP information.
(Note: Store the files to C:\inetpub\wwwroot. If you have to store the files in a separate folder, then change the path in system variables.)
Thursday, July 16, 2009
Facebook Reaches 250 Million Users
window.google_render_ad();
"From the beginning, Facebook hasn't been about building a website. Facebook is about all of the people using it and all of the things that are important to you. The 250 million of you on Facebook today are what gives Facebook life and makes the site meaningful to everyone using it, so we thank you," co-founder and CEO Mark ZUckerberg wrote. "Each person who joins makes Facebook better by adding a presence to the site that friends and family can connect with and feel closer to. For us, growing to 250 million users isn't just an impressive number; it is a mark of how many personal connections all of you have made, and how far we at Facebook have to go to extend the power of connection to the billions of people around the world."More surprising than the raw number is perhaps the rate at which Facebook is acquiring them, at 700,000 to 750,000 new users per day in the recent weeks, according to InsideFacebook, though, as a rough estimate, considering it's been a little over three months since the company announced it had reached 200 million users, the number would come to an average of 500,000 per day. Most of the growth comes from outside the US, roughly 70 percent of it, but it also managed to consolidate its position as the number one social network in the States too. With the announcement of the 250 million milestone came a revised stats page with some interesting information because, not only is it the biggest social network, it also has some of the most engaged users with 120 million logging in at least once a day and 30 million updating their statuses at least daily. A recent study also showed that it was the most visited site in the US with users spending 4 hours and 39 minutes on average every month.